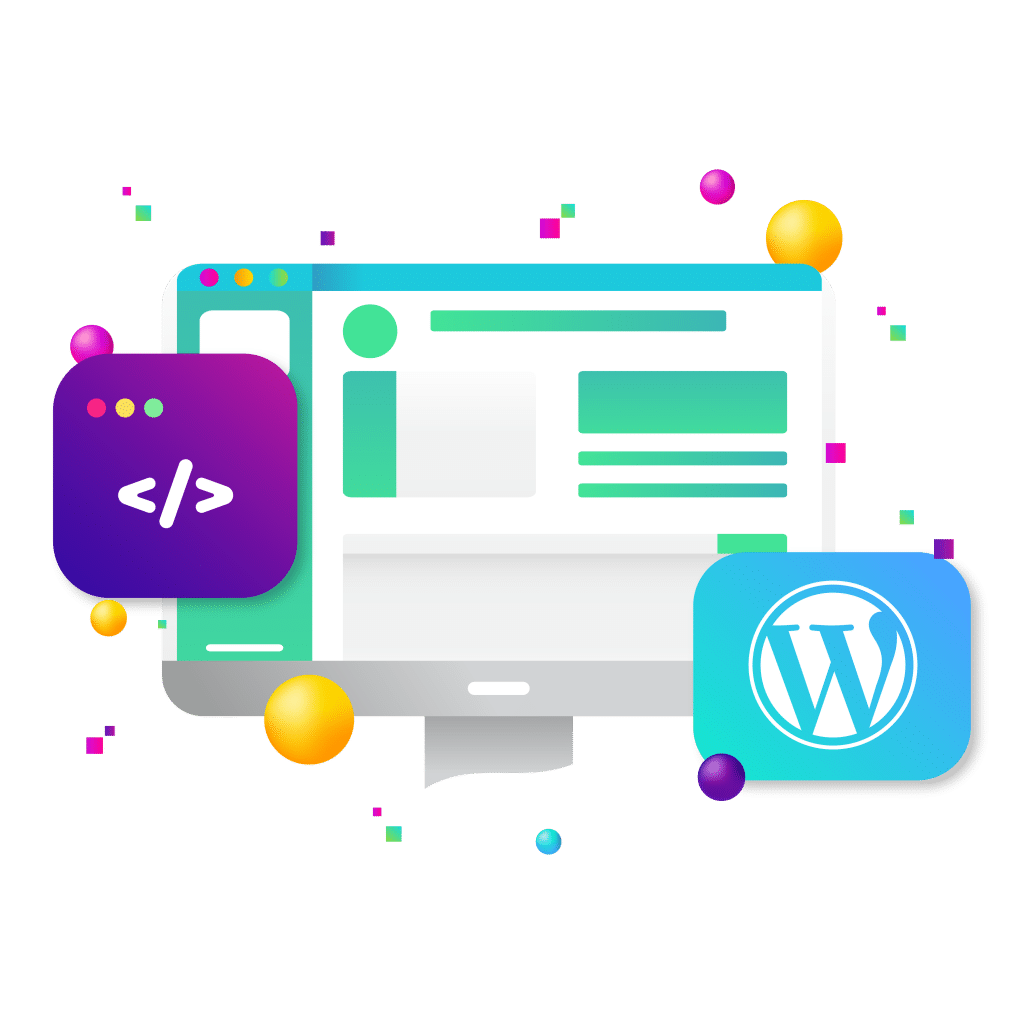
Can I build a custom React frontend for my WordPress site? This question is increasingly common among developers aiming to merge the robust content management capabilities of WordPress with the dynamic, responsive user interfaces that React offers. By combining WordPress with React, you can create fast, interactive experiences without sacrificing the ease of content management that WordPress provides. In this guide, we’ll walk you through the process of developing a custom React frontend that interacts seamlessly with your WordPress backend, unlocking the full potential of both platforms.
Why Combine WordPress with React?
Benefits of WordPress
WordPress is a powerful and flexible content management system (CMS) that powers over 40% of websites worldwide. Its user-friendly interface allows for easy content creation and management, even for non-technical users. With a vast library of plugins and themes, WordPress can be customized to fit a wide range of needs, from simple blogs to complex e-commerce sites. Its large community provides extensive support and resources, making it a reliable choice for web development.
Advantages of React
React is a popular JavaScript library developed by Facebook for building user interfaces. It excels at creating dynamic, high-performing, and responsive frontends. React’s component-based architecture promotes reusability and maintainability, allowing developers to build complex interfaces efficiently. With React, you can create seamless user experiences that feel like desktop applications, thanks to its efficient rendering and state management.
Why They Complement Each Other
Combining WordPress with React allows you to use WordPress as a headless CMS, where it handles the backend data and content management, while React takes care of rendering the frontend. This approach leverages the strengths of both platforms: WordPress’s ease of content management and React’s flexibility in building modern, responsive user interfaces. By decoupling the frontend and backend, you gain greater control over the user experience and can create highly customized, interactive websites.
How React Integrates with WordPress
Introduction to Headless WordPress
A headless WordPress setup involves separating the frontend presentation layer from the backend content management system. In this configuration, WordPress serves as a backend API that provides data to any frontend application via REST API or GraphQL. This means you can use React, or any other frontend technology, to consume WordPress data and render it as you see fit.
WordPress REST API
The WordPress REST API is a powerful feature that allows developers to interact with WordPress data using HTTP requests. It provides endpoints for retrieving and manipulating content like posts, pages, and custom post types in JSON format. This makes it straightforward for React applications to fetch and display WordPress content.
GraphQL Option with WPGraphQL
WPGraphQL is a free, open-source WordPress plugin that adds a GraphQL API to your WordPress site. GraphQL allows clients to request exactly the data they need, reducing the amount of data transferred and improving performance. With WPGraphQL, you can create more efficient and flexible queries compared to the REST API.
REST API vs. WPGraphQL
While both the REST API and WPGraphQL enable data retrieval from WordPress, they have some differences:
- REST API: Uses predefined endpoints and returns all data associated with that endpoint, which can sometimes result in over-fetching.
- WPGraphQL: Allows you to specify exactly what data you need in your queries, reducing unnecessary data transfer and improving performance.
Your choice between them depends on your project’s specific needs and your familiarity with each technology.
Setting Up Your WordPress Backend for React
Ensuring API Access
To prepare your WordPress site for integration with React, you need to ensure that the necessary APIs are available:
- For REST API: It’s built into WordPress core, so no additional setup is required.
- For WPGraphQL: Install and activate the WPGraphQL plugin from the WordPress plugin repository.
Enabling Headless Mode
Since you’ll be using React for the frontend, you might want to disable the default WordPress frontend:
- Use a Barebones Theme: Install a minimal theme that doesn’t output any frontend code, such as “BlankSlate” or “UnderStrap.”
- Disable Frontend Rendering: Use plugins like “Disable Gutenberg” or custom code to prevent WordPress from rendering the frontend.
Securing API Endpoints
Security is paramount when exposing your WordPress data via APIs:
- Authentication: Implement authentication methods like OAuth 2.0 or JSON Web Tokens (JWT) to secure your endpoints.
- Permissions: Set proper user roles and capabilities to control access to sensitive data.
- HTTPS: Ensure your site uses SSL/TLS to encrypt data in transit.
Building the React Frontend
Creating a React App
Set up your React application using tools like Create React App for a single-page application or Next.js for server-side rendering (SSR) and static site generation (SSG):
# Create a new React application using Create React App
# This command will generate a new project folder called 'my-react-app'
npx create-react-app my-react-app
# Create a new Next.js application using Create Next App
# This command will generate a new project folder called 'my-react-app' using Next.js
npx create-next-app my-react-app
Fetching Data from WordPress
Use the fetch API or Axios to retrieve data from the WordPress REST API or WPGraphQL:
// Using fetch to get posts from WordPress REST API
fetch('https://your-wordpress-site.com/wp-json/wp/v2/posts')
.then(response => response.json()) // Convert the response to JSON
.then(posts => {
// Handle the posts data
console.log(posts); // Output the posts to the console
})
.catch(error => console.error('Error fetching posts:', error)); // Handle any errors
// Using Apollo Client for WPGraphQL to get posts
import { ApolloClient, InMemoryCache, gql } from '@apollo/client';
// Initialize Apollo Client
const client = new ApolloClient({
uri: 'https://your-wordpress-site.com/graphql', // GraphQL endpoint for the WordPress site
cache: new InMemoryCache() // In-memory cache for the Apollo client
});
// Fetch posts using a GraphQL query
client
.query({
query: gql`
query GetPosts {
posts {
nodes {
id // Post ID
title // Post title
content // Post content
}
}
}
`
})
.then(result => console.log(result)) // Output the result to the console
.catch(error => console.error('Error fetching posts:', error)); // Handle any errors
Managing State in React
For state management in your React application:
- React Hooks: Utilize useState and useEffect for local state and side effects.
- Context API: Manage global state without prop drilling.
- Redux: Use for more complex state management needs.
Displaying Content from WordPress
Create components to display your WordPress content:
// Post.js
import React from 'react';
// Component to display a single post
function Post({ title, content }) {
return (
{/* Render post title and content, allowing HTML rendering via dangerouslySetInnerHTML */}
);
}
export default Post;
Best Practices for WordPress with React Integration
Performance Optimization
- Lazy Loading: Use React.lazy() and Suspense to load components only when needed.
- Code Splitting: Implement code splitting to reduce initial load times.
- Server-Side Rendering (SSR): Use Next.js for SSR to improve performance and SEO.
SEO Considerations
SEO can be challenging with client-side rendering:
- Use SSR or SSG: Frameworks like Next.js can render pages on the server or at build time.
- Meta Tags Management: Use libraries like react-helmet or Next.js’s Head component to manage meta tags.
Security
- Protect API Routes: Secure your WordPress endpoints to prevent unauthorized access.
- Input Validation: Always validate and sanitize user inputs in forms.
- Update Regularly: Keep WordPress, plugins, and your React dependencies up to date.
Advanced Techniques and Customizations
Custom Post Types and Fields
To handle more complex data structures:
- Advanced Custom Fields (ACF): Add custom fields to your WordPress content.
- Expose ACF to APIs: Use plugins like “ACF to REST API” or ensure WPGraphQL supports your custom fields.
WordPress Plugins for React Integration
- WPGraphQL: Provides a GraphQL API for WordPress.
- ACF: For creating custom fields.
- WP REST API Menus: Exposes WordPress menus via the REST API.
Handling User Authentication and Interactions
Implement authentication to handle user-specific content:
- JWT Authentication: Use the “JWT Authentication for WP REST API” plugin.
- OAuth 2.0: Set up OAuth authentication for more secure flows.
When to Consider Headless WordPress
- Need for High Performance: When site speed and user experience are top priorities.
- Complex Frontend Functionality: When building applications that require advanced interactivity.
- Multi-Channel Content Distribution: When serving content to various platforms beyond the web.
Common Pitfalls
- Increased Development Complexity: Requires knowledge of both React and WordPress APIs.
- Maintenance Overhead: Managing two separate codebases can be more demanding.
- SEO Challenges: Client-side rendering may not be optimal for SEO without proper configurations.
By combining React with WordPress, you can create dynamic, high-performing websites that offer exceptional user experiences while maintaining the powerful content management capabilities of WordPress. Whether you’re building a complex web application or a simple blog with interactive features, integrating WordPress with React opens up a world of possibilities.
New Target can elevate your business by seamlessly integrating WordPress with React, providing a powerful, dynamic digital presence. Whether you are a company, an association, or a government agency, New Target’s deep expertise in WordPress design and engineering becomes your competitive advantage. By utilizing React for creating fast, interactive front-end experiences and combining it with the robust back-end of WordPress, we don’t just build websites—we craft a digital narrative tailored to amplify your brand’s message and deliver the results you need.Â